Wynn O’Donnell
IDM 382: Internet of Things
Fall 2020
For this project, I worked on developing an Arduino Piano with sound that can be modified and played through a browser. I called the original product in West107 the Arduorgan, a combination of the word Arduino, and the word Organ, a large piano-like musical instrument. If I go through with that theme this project will be called the Arduynthesizer, as it is an Arduino synthesizer. The Arduynthesizer will send signals to a browser when a button is pressed on it, and the browser will produce a sound based on the settings selected in the browser. The user will control the Arduynthesizer by pressing buttons on the Arduino, and by changing settings in a browser window with sliders. These sliders will include Pitch, volume, and tone.
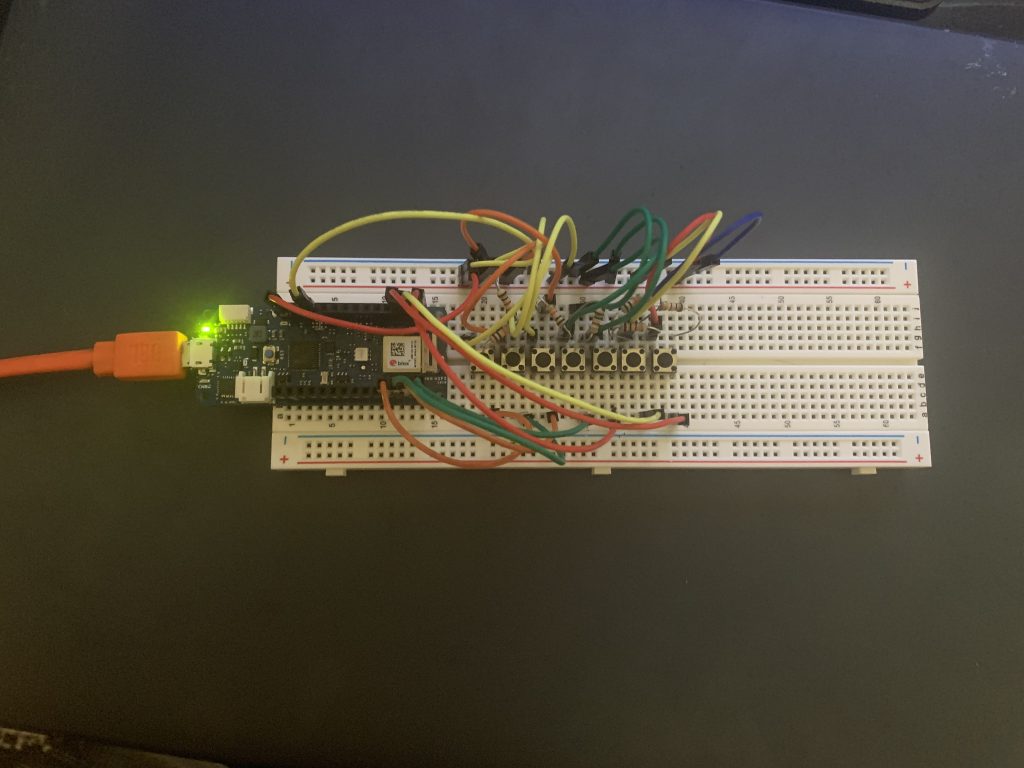
Goals
- Add more features to an Arduino piano I created in West107
- Make it more like a synthesizer
- Add variation to the sounds it can produce
- Make the sound to be able to be controlled and heard from a browser window
Final Presentation
Sample Code
/* #include "thingProperties.h" const int buttonPin1 = 2; const int buttonPin2 = 3; const int buttonPin3 = 4; const int buttonPin4 = 5; const int buttonPin5 = 6; const int buttonPin6 = 7; const int buttonPin7 = 8;// the number of the pushbutton pin const int ledPin = 13; // the number of the LED pin int buttonState1 = 0; int buttonState2 = 0; int buttonState3 = 0; int buttonState4 = 0; int buttonState5 = 0; int buttonState6 = 0; int buttonState7 = 0; String buttonpressStr1 = "Play C Note"; String buttonpressStr2 = "Play D Note"; String buttonpressStr3 = "Play E Note"; String buttonpressStr4 = "Play F Note"; String buttonpressStr5 = "Play G Note"; String buttonpressStr6 = "Play A Note"; String buttonpressStr7 = "Play B Note"; void setup() { // Defined in thingProperties.h initProperties(); // Initialize serial and wait for port to open: Serial.begin(9600); // This delay gives the chance to wait for a Serial Monitor without blocking if none is found delay(1500); // initialize the LED pin as an output: pinMode(ledPin, OUTPUT); // initialize the pushbutton pin as an input: pinMode(buttonPin1, INPUT); pinMode(buttonPin2, INPUT); pinMode(buttonPin3, INPUT); pinMode(buttonPin4, INPUT); pinMode(buttonPin5, INPUT); pinMode(buttonPin6, INPUT); pinMode(buttonPin7, INPUT); // Connect to Arduino IoT Cloud ArduinoCloud.begin(ArduinoIoTPreferredConnection); /* The following function allows you to obtain more information related to the state of network and IoT Cloud connection and errors the higher number the more granular information you’ll get. The default is 0 (only errors). Maximum is 4 */ setDebugMessageLevel(2); ArduinoCloud.printDebugInfo(); } void loop() { ArduinoCloud.update(); // Your code here buttonState1 = digitalRead(buttonPin1); buttonState2 = digitalRead(buttonPin2); buttonState3 = digitalRead(buttonPin3); buttonState4 = digitalRead(buttonPin4); buttonState5 = digitalRead(buttonPin5); buttonState6 = digitalRead(buttonPin6); buttonState7 = digitalRead(buttonPin7); // check if the pushbutton is pressed. If it is, the buttonState is HIGH: if (buttonState1 == HIGH) { // turn LED on: digitalWrite(ledPin, HIGH); Serial.println("Play C Note"); messenger = buttonpressStr1; } else { // turn LED off: digitalWrite(ledPin, LOW); } if (buttonState2 == HIGH) { // turn LED on: digitalWrite(ledPin, HIGH); Serial.println("Play D Note"); messenger = buttonpressStr2; } else { // turn LED off: digitalWrite(ledPin, LOW); } if (buttonState3 == HIGH) { // turn LED on: digitalWrite(ledPin, HIGH); Serial.println("Play E Note"); messenger = buttonpressStr3; } else { // turn LED off: digitalWrite(ledPin, LOW); } if (buttonState4 == HIGH) { // turn LED on: digitalWrite(ledPin, HIGH); Serial.println("Play F Note"); messenger = buttonpressStr4; } else { // turn LED off: digitalWrite(ledPin, LOW); } if (buttonState5 == HIGH) { // turn LED on: digitalWrite(ledPin, HIGH); Serial.println("Play G Note"); messenger = buttonpressStr5; } else { // turn LED off: digitalWrite(ledPin, LOW); } if (buttonState6 == HIGH) { // turn LED on: digitalWrite(ledPin, HIGH); Serial.println("Play A Note"); messenger = buttonpressStr6; } else { // turn LED off: digitalWrite(ledPin, LOW); } if (buttonState7 == HIGH) { // turn LED on: digitalWrite(ledPin, HIGH); Serial.println("Play B Note"); messenger = buttonpressStr7; } else { // turn LED off: digitalWrite(ledPin, LOW); } }//End loop void onMessengerChange() { // Do something }